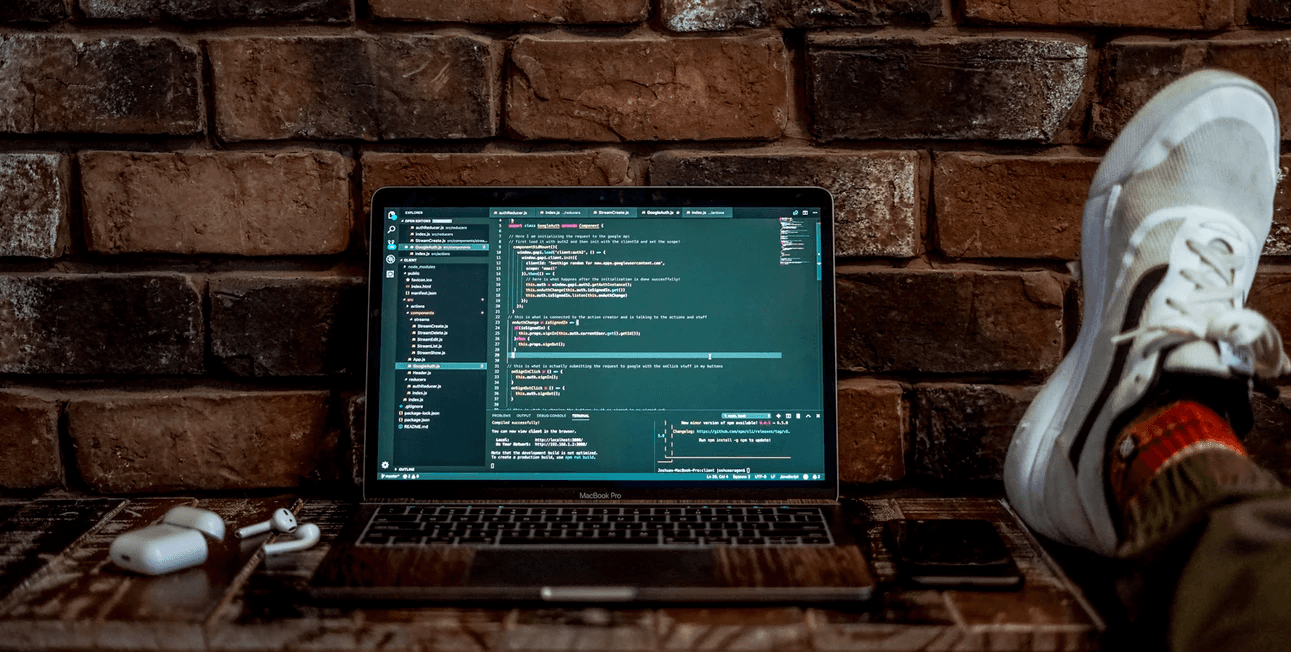
Documenting Play Framework with Swagger
Timur Isachenko
Documenting Play Framework with Swagger
Swagger makes API documentation and exploration a breeze, offering a variety of tools for the entire API development lifecycle, from design and testing to deployment. If you’re working with the Play Framework, here’s a detailed guide to integrating Swagger for dynamic, auto-generated API documentation.
Introduction to Play Framework
Play Framework is an MVC web framework for building modern web applications in Java and Scala. Developed by Lightbend, it emphasizes developer productivity and reactive programming principles.
- Official Website: Play Framework
- Play Framework on Wikipedia: Play (framework)
Introduction to Swagger
Swagger (now part of the OpenAPI Initiative) is a set of tools for designing, building, and documenting APIs. The most famous tools include Swagger UI and Swagger Codegen.
- Official Website: Swagger
- Wikipedia Page: Swagger (software)
Setting Up API Documentation with Swagger
Here’s how to integrate Swagger with Play Framework for automatic API documentation. We’ll use Play Framework 2.6.3 as an example.
Step 1: Add the Swagger Module
- Add the
swagger-play2
dependency in yourbuild.sbt
file:
libraryDependencies += "io.swagger" %% "swagger-play2" % "1.6.0"
- Enable the Swagger module in
conf/application.conf
:
play.modules.enabled += "play.modules.swagger.SwaggerModule"
api.version = "v1" // Specify the API version
For additional configurations, refer to the swagger-play2 documentation.
Step 2: Documenting API Endpoints
- Add Swagger annotations: Use the
io.swagger.annotations
package to annotate your controller classes and methods.
Example:
@Api(value = "User Controller", produces = "application/json")
public class UserController extends Controller {
@ApiOperation(value = "Get User", notes = "Fetch user details by ID", response = User.class)
@ApiResponses({
@ApiResponse(code = 404, message = "User not found"),
@ApiResponse(code = 500, message = "Internal server error")
})
public Result getUser(@ApiParam(value = "User ID", required = true) String userId) {
User user = userService.getUser(userId);
return ok(Json.toJson(user));
}
}
Step 3: Add Routes for Swagger Spec
Expose your Swagger JSON specification by adding the following route in conf/routes
:
GET /docs/swagger.json controllers.ApiHelpController.getResources
Now, the Swagger specification will be available at /docs/swagger.json
.
Step 4: Adding Swagger UI
Swagger UI is a frontend for visualizing and interacting with your API documentation.
- Download the Swagger UI distribution and place it in your Play project's
public/swagger-ui
directory. - Configure routes to serve the Swagger UI:
GET /docs/ controllers.Assets.at(path="/public/swagger-ui", file="index.html")
GET /docs/swagger.json controllers.ApiHelpController.getResources
GET /docs/*file controllers.Assets.at(path="/public/swagger-ui", file)
- Update the
index.html
file in the Swagger UI directory to point to your Swagger JSON spec:
var url = "swagger.json";
Step 5: Testing the Setup
Run your Play Framework project using sbt run
and navigate to http://localhost:9000/docs/
. You should see the Swagger UI displaying your API documentation dynamically.
Pros and Cons of Swagger Integration
Advantages:
- Improved API Communication: Automatically generated documentation simplifies communication with API consumers.
- Code Generation: Swagger can generate client SDKs in various languages, reducing development time.
- Interactive UI: Enables developers and testers to experiment with API endpoints directly.
Limitations:
- Swagger UI can occasionally require manual page reloads to display updates.
- If the API service is unavailable, Swagger UI does not provide clear error messages.
- API path prefixes are not handled automatically in Swagger UI.
- Security configuration in Swagger spec generation is not fully mature.
- Play Framework support for Swagger may lag for newer Play versions.
Conclusion
Swagger is an excellent tool for documenting and interacting with APIs. With its dynamic UI and ease of integration, it’s a must-have for any modern API project. While it has minor limitations, its benefits in terms of communication, testing, and client generation far outweigh its drawbacks.
For further reference, check out this video tutorial on integrating Swagger with Play Framework.
Happy coding! 🎉